5 Python tricks I learned from Advent of Code 2022
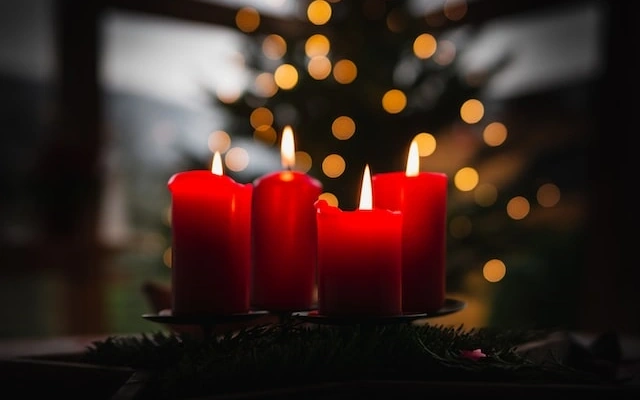
Table of Contents
As someone who loves to practice code optimization techniques, December has become one of my favourite times of the year. Like last year, I participated in Advent of Code. This is an annual set of Christmas-themed programming puzzles that follow an advent calendar. This is the second time I participated, because it is a great way to practice my coding skills on problems I do not normally encounter.
One of my favourite things about it is that everyone is sharing their solutions on reddit or other forums. I love to share my own tricks, and even more to read about how others approached the same problem. I often go back to improve my own solutions to implement what I learned.
This is a short list of some of the tricks that I picked up this year.
Count #
Many puzzles require you to keep of some round or iteration number. While you could manually increment n
in a while-loop, you can also iterate over count()
. I used this in
day 23 and
day 24.
|
|
This is especially useful to factor out the loop for some solutions. For day 23 I used this pattern to re-use the same code for both part 1 and 2:
|
|
Divmod #
Divmod is a python builtin that does division and modulo in a single function. It literally returns the (x//y
, x%y
):
|
|
Cool! I used this in day 17 and 25 to save a few lines of code.
Complex numbers are magic #
Complex numbers can be used to store coordinates. Who knew!? I saw many solutions using this trick in combinations with sets hack to avoid having to set up a custom Pointer(x, y)
class or (x, y)
tuples. Where the real and imaginary components represent the x and y coordianates.
Complex numbers have 3 distinct advantages:
- Complex numbers are immutable, so they can be added to sets (tuples or dataclasses cannot).
|
|
- They are a builtin type. This makes using them in list comprehensions a breeze.
|
|
- Complex math is really interesting for coordinate systems. Complex numbers are almost a coordinate system in itself. For example, a clockwise rotation is simply a multiplication by
-1j
:
|
|
After seeing some solutions use this I tried it myself during
day 14. While it made the code shorter, I thought it was a bit challenging to reason about it for myself. I tried again in some later solutions, but I prefer to pass row and column ((r, c)
) tuples.
Sets are useful as sparse matrices #
As someone with a background in image processing, I immediately reach for numpy
whenever something can be parsed into an array. This turned out to be the wrong choice on
day 14 and
23.
In both cases, the array could be infinitely expanded which made numpy the wrong choice. In fact, in both puzzles, you could simply keep track of the ‘blocked’ positions as a set of coordinates. Bonus: checking whether coordinates are in a set is really fast in Python.
For example, this snippet from my solution for day 24:
|
|
for/else #
for/else
and while/else
are odd statements in Python. If it were up to Guido,
he would not have included them at all. Their use is highly discouraged, because it’s somewhat confusing what to do. In my head I read it as for/nobreak
. However, they can be useful on some occasions. This advent of code I found two perfect opportunities to use them.
|
|
For example, I used this in my solutions for day 14:
|
|
and day 23:
|
|
Conclusion #
There you have it, some of the tricks I picked up during advent of code. See you next year! 🎄