My 5 favourite IPython tricks
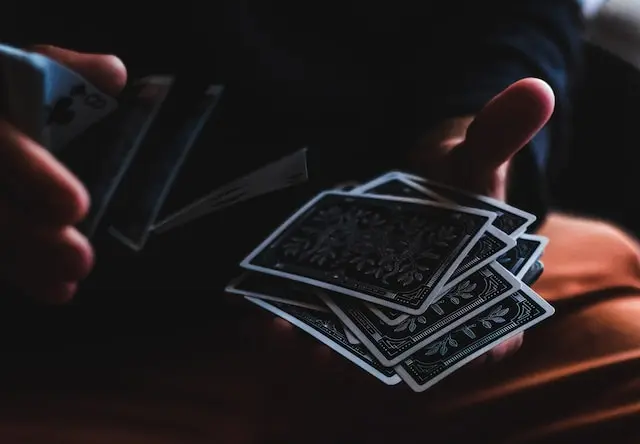
Table of Contents
These are my 5 favourite tricks I use daily when developing code using IPython, either in the shell or via a Jupyter notebook.
Auto reload #
My all time favourite IPython magic is the auto reload function. This is an extension that automatically reloads modules before executing a cell. Whenever I’m working on a library, I add these 2 lines at the top of my notebook. This means that whenever I change the library I’m working, without having to restart the kernel. Super convenient for debugging.
|
|
Timing #
For some quick performance testing of benchmarking, you can use the built-in time magics.
There are cell magics to time (%%time
) or benchmark (%%timeit
) the cell. Or you can time (%time
) and (%timeit
) a single line or function call.
Add virtual environment as Jupyter kernel #
One of my habits is to create a custom virtual environment for every code that I’m working on. These are usually called .venv
, and I want to enable these as a kernel in a Jupyter Notebook.
I do this so infrequently that I can never remember the command:
|
|
Breakpoint #
I use the breakpoint()
function added in Python 3.7 all the time when developing code. Before that I was already calling the IPython.embed
module directly, but using a breakpoint()
statement is much more convenient.
Especially, because you can customize the debugger by setting the PYTHONBREAKPOINT
environment variable. The snippet below will open an IPython shell instead of the default pdb
by:
|
|
Or in your .bashrc
:
|
|
Sublime keybindings in Jupyter Notebook #
As a daily Sublime Text user, the ctrl-D command to select the next word is burned into my fingers. One of my annoyances with the default install of Jupyter is that ctrl-D deletes the current line. There are different ways to achieve this ways to enable the Sublime keymap in a Jupyter environment. I found this way to be the most reliable.
Copy this snippet into ~/.config/jupyter/custom/custom.js
|
|