- Stef Smeets - Research Software Engineer/
- Posts/
- TIL you can refactor your documentation using decorators/
TIL you can refactor your documentation using decorators
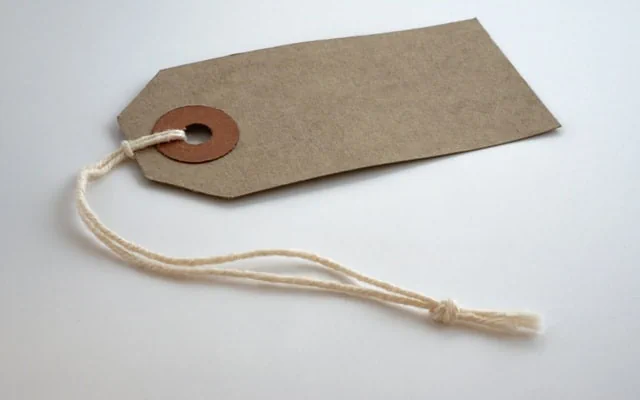
I don’t mind writing documentation. I try to write and update docstrings as I’m working on the code. This is not always easy, and sometimes documentation is spread over different docstrings. Or if docstrings for similar functions are not consistent. This, I do mind. Especially if they are part of the public API.
Last week I found that you can copy docstrings using a decorator. This post is inspired by some code I found in matplotlib:
|
|
It’s a decorator for a function, that copies the docstring from the source function to the decorated function.
|
|
Help on function roar in module __main__:
roar()
Roar like a lion.
Help on function loud_roar in module __main__:
loud_roar()
Roar like a lion.
Cool! Not immediately useful for my own purposes. The good thing is that it can easily be extended using string formatting.
The decorator below takes a function, and tries to copy the docstring to the target function. Any keyword parameters are passed to string.formatting
. A copy of the source docstring is kept on ._docstring
.
|
|
Let’s see how it works:
|
|
Help on function roar in module __main__:
roar()
Roar like a cow.
Help on function moo in module __main__:
moo()
Moo like a cow.
It also works with class methods:
|
|
Help on function min in module __main__:
min(self)
Return minimum value from the list.
Help on function max in module __main__:
max(self)
Return maximum value from the list.
Help on function mean in module __main__:
mean(self)
Return median value from the list.
Essentially anything with a docstring (__doc__
attribute) works, such as classes:
|
|
This is A.
This is B.
Nice! you can imagine that if you have large docstrings, that helps keeping the documentation consistent and concise.
As a bonus, this will also be picked up by Sphinx. If you are generating documentation for e.g. readthedocs, this will make it just a little bit nicer to read!