Streamlit + PySide = standalone data app
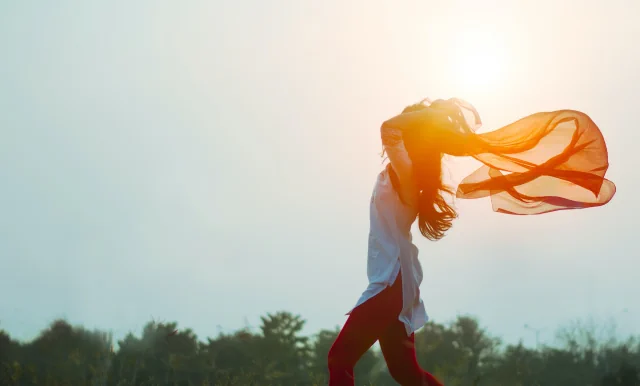
Table of Contents
For one of my projects I wanted to be able to open a streamlit app in its own window. Streamlit is an excellent way to turn scripts into web apps that I like to use to showcase research data. By default, it starts a web server and attempts to open this url in a webbrowser.
I was curious if it would be possible to redirect this to some sort of Python webview, so that I could turn my dashboard into a standalone app.
Qt webview #
It turns out that Qt (using PySide2) has this capability via the QtWebEngineWidgets
. The most straightforward way to install is via conda:
|
|
With pyside
installed, it is straightforward enough to make a little window pop up showing any url:
|
|
This is what it looks like:
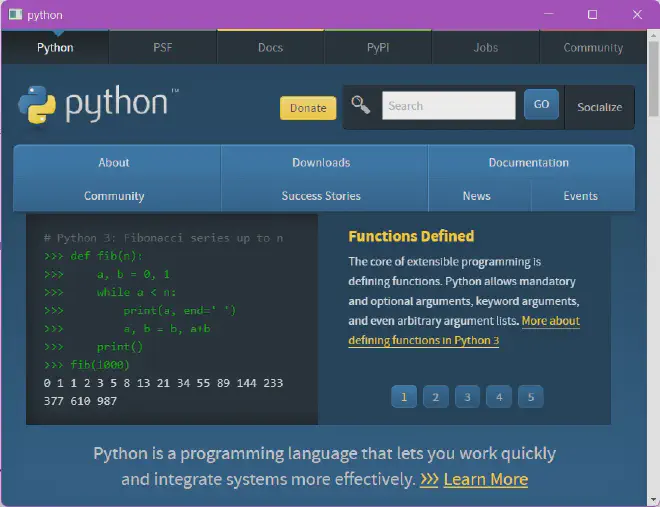
Wrapping streamlit #
To start a streamlit server (and closing it!) is a bit more involved. Normally you would run streamlit run my_script.py
, and then pop the url into your webbrowser. But somehow we must wrap this behaviour. This is what I ended up with:
|
|
- In this example I use
streamlit hello
. You can exchange this with the path to your script. - For the streamlit command, we need
--server.headless=True
. This will prevent streamlit from trying to open the URL in your default browser. - I run the streamlit command in subprocess. This is to isolate the event loop. I tried going with threads, but using a subprocess is so much easier.
- Therefore, we must kill the streamlit server when we close the webview. To do so we can use the
atexit
handler. On Linux we can usep.kill()
, but on Windows we must send thetaskkill
command. From experience,p.kill()
is not adequate on Windows.
This is the end result:
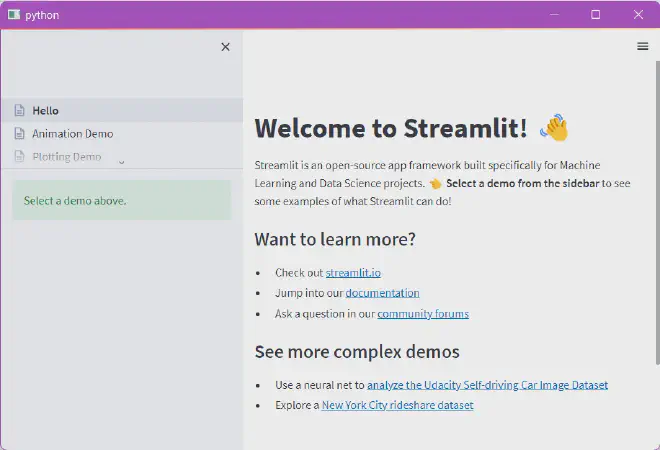
No browser required :-)